Cameron Fowler recommends you simplify your toolset by replacing Postman with Bash
Postman is a great API testing tool. It has a boat-load of functionality that I will never use, but at the heart of it, it does these things:
-
Calls an API in a reproducible way
-
Formats the response nicely
-
Handles passing variables into the API request and parsing variables out of the API response
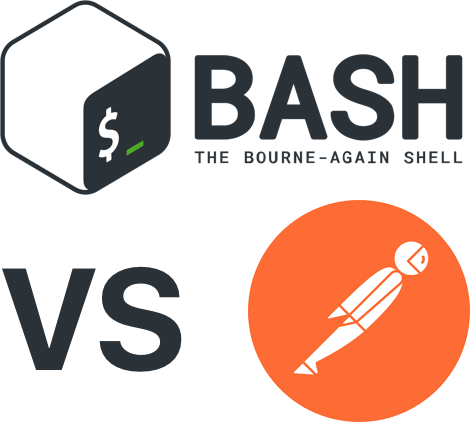
I’m all in favour for up-skilling in tools I already have available on my machine, and simplifying my toolset. Often I find that some product I’ve been using for years is actually solving a problem that has already been solved by a developer in the form of a command line tool.
I figured that this is a fairly simple problem to solve using bash
and curl
and some JSON parsing (in my case, I used jq
). It turns out it is simple and very powerful.
Simply set some environment variables in a file:
# file: ./caller/cameron
export HOST="my.test.example.com"
export USERNAME="cameron"
export password="..."
Then put other HTTP calls in other files, and source them from the command line. I would expect to call our ‘system’ like this:
. caller/cameron # which container the above environment variables
. get_bearer_token # which calls an API and gets a token, setting it as another environment variable
./get_test_endpoint # a simple authorised API call
./post_test_endpoint # an example of a post.
An example of how you might use the response to get out a BEARER token:
# file: ./get_bearer_token
curl --trace-ascii last_trace.curl \
--include --silent --show-error \
--header [@headers](http://twitter.com/headers) \
--request POST \
--url "$HOST/auth/token" \
--data @- <<EOF > last_response.http
{
"username": "$USERNAME",
"password": "$PASSWORD",
"clientId": "test-client",
"grantType": "password",
"remember": false
}
EOF
cat last_response.http
echo
BEARER_TOKEN="$(tail -n 1 last_response.http | jq -r '.accessToken')"
export BEARER_TOKEN
echo "$BEARER_TOKEN"
A GET:
file: ./get_test_endpoint (dont forget chmod +x)
curl --trace-ascii last_trace.curl \
--include --silent --show-error \
--header "Authorization: Bearer $BEARER_TOKEN" \
--request GET \
--url "$HOST/test-endpoint" > last_response.http
A cut down POST example:
file: ./post_test_endpoint (dont forget chmod +x)
curl --trace-ascii last_trace.curl \
--include --silent --show-error \
--header "Authorization: Bearer $BEARER_TOKEN" \
--request POST \
--url "$HOST/test-create-endpoint" \
--data @- <<EOF > last_response.http
{
"mode": "test",
"payload": null,
"testdata": 0,
"easyJSON": true
}
EOF